本篇文章接着上一篇最后的程序进行了增加和改写,实现了利用MyBatis框架对数据的增删改查。本次测试方法有所不同,没有在main方法下执行而是利用了@Test注解,自己导一下库,然后可以通过右键Run as ->JUnit Test来进行测试。然后就是一直有个小问题,就是日志一直不显示在控制台,我查了也不好使,将来再进行更改。
数据库测试前数据库数据如图:
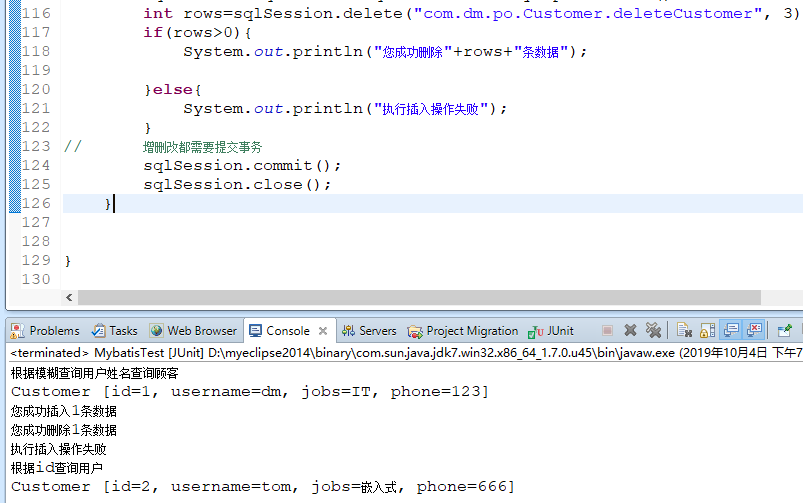
JUnit信息:
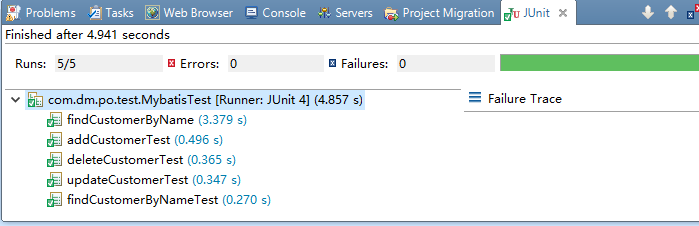
数据库数据:
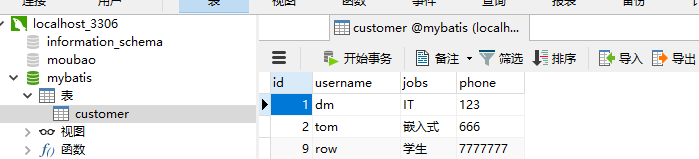
接下来附上源代码:
首先还是项目结构:
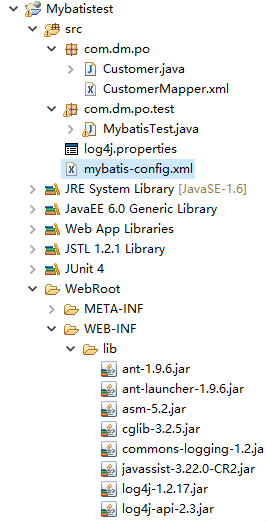
Customer.java
1 | package com.dm.po; |
CustomerMapper.xml
1 |
|
MybatisTest.java
1 | package com.dm.po.test; |
log4j.properties
1 | # Global logging configuration |
mybatis-config.xml
1 |
|