二叉排序树及二叉树常见算法
一、定义
二叉排序树,又叫二叉查找树,它或者是一棵空树;或者是具有以下性质的二叉树:
若它的左子树不空,则左子树上所有节点的值均小于它的根节点的值;
若它的右子树不空,则右子树上所有节点的值均大于它的根节点的值;
它的左右子树也分别为二叉排序树。
如下图:
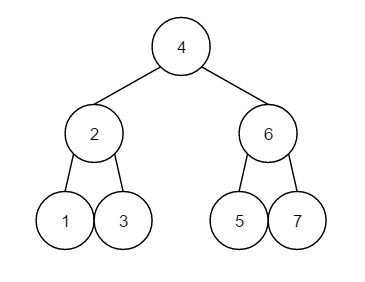
二、基本方法
1 | //定义 |
输出结果:
三、如何层序遍历二叉树
可以使用队列来实现二叉树的层序遍历。其主要思想如下:现将根结点放在队列中,然后每次都从队列中取出一个结点打印打印该结点的值,若这个结点有子结点,则将他的子结点放在队列尾,直到队列为空。
1 | //层序遍历 |
四、 二叉树的最大深度
1 | public int maxDepth(TreeNode root) { |
五、二叉树的镜像
1 | public void Mirror(TreeNode root) { |
六、 对称二叉树
1 | boolean isSymmetrical(TreeNode pRoot){ |
七、路径总和
1 | public class Solution { |
八、 重建二叉树
1 | public TreeNode reConstructBinaryTree(int [] pre,int [] in) { |
九、 二叉搜索树的后序遍历序列
public boolean VerifySquenceOfBST(int [] sequence) {
if(sequence.length==0)
return false;
int len = sequence.length-1;
return split(sequence,0,len);
}
public boolean split(int [] sequence,int start,int end){
if(start>=end)
return true;
int center = start;
while(sequence[center]<sequence[end]&¢er<end){
center++;
}
for(int i=center;i<end;i++){
if(sequence[i]<sequence[end]){
return false;
}
}
return split(sequence,start,center-1)&&split(sequence,center,end-1);
}
十、二叉树的序列化和反序列化
1 | public String serialize(TreeNode root) { |
反序列化:
1 | public TreeNode deserialize(String data) { |